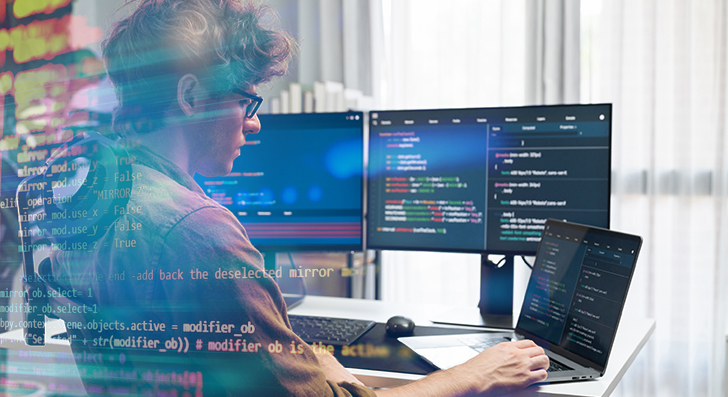
Scalability usually means your application can handle advancement—additional consumers, much more details, plus more visitors—without breaking. For a developer, constructing with scalability in mind will save time and tension afterwards. Listed here’s a clear and realistic guidebook to help you start out by Gustavo Woltmann.
Design and style for Scalability from the beginning
Scalability isn't really a thing you bolt on later—it ought to be component within your program from the start. Several purposes fail if they improve quick for the reason that the original style and design can’t deal with the additional load. As a developer, you must Imagine early regarding how your system will behave under pressure.
Start by planning your architecture to generally be flexible. Prevent monolithic codebases where almost everything is tightly related. As a substitute, use modular design or microservices. These designs split your application into smaller, independent areas. Each individual module or services can scale on its own with out impacting The full procedure.
Also, think about your database from day one particular. Will it have to have to handle 1,000,000 people or just a hundred? Choose the proper variety—relational or NoSQL—based upon how your details will grow. Strategy for sharding, indexing, and backups early, Even when you don’t have to have them yet.
Yet another critical place is to stop hardcoding assumptions. Don’t generate code that only works under present problems. Contemplate what would transpire In the event your person foundation doubled tomorrow. Would your application crash? Would the database slow down?
Use style patterns that assistance scaling, like concept queues or function-driven methods. These assist your app handle more requests without the need of having overloaded.
After you Develop with scalability in mind, you are not just planning for achievement—you're decreasing future problems. A very well-planned technique is simpler to maintain, adapt, and develop. It’s better to arrange early than to rebuild later on.
Use the proper Databases
Picking out the appropriate database is really a key Element of constructing scalable programs. Not all databases are built the exact same, and using the wrong you can slow you down or even cause failures as your application grows.
Commence by understanding your facts. Is it really structured, like rows in the table? If Of course, a relational database like PostgreSQL or MySQL is a great healthy. These are generally strong with associations, transactions, and consistency. Additionally they support scaling approaches like study replicas, indexing, and partitioning to take care of much more traffic and facts.
In case your details is much more adaptable—like user action logs, product catalogs, or paperwork—take into consideration a NoSQL alternative like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at managing big volumes of unstructured or semi-structured knowledge and will scale horizontally a lot more quickly.
Also, think about your read through and write patterns. Will you be doing many reads with fewer writes? Use caching and read replicas. Will you be managing a hefty publish load? Take a look at databases that can manage significant generate throughput, as well as party-based information storage programs like Apache Kafka (for momentary details streams).
It’s also intelligent to Feel forward. You might not have to have advanced scaling characteristics now, but deciding on a database that supports them indicates you won’t want to change later on.
Use indexing to speed up queries. Keep away from unwanted joins. Normalize or denormalize your data depending on your entry designs. And constantly keep an eye on databases effectiveness while you increase.
Briefly, the appropriate databases will depend on your application’s composition, velocity demands, And just how you assume it to expand. Get time to pick wisely—it’ll save loads of hassle afterwards.
Improve Code and Queries
Rapid code is vital to scalability. As your app grows, each small hold off provides up. Badly created code or unoptimized queries can slow down general performance and overload your process. That’s why it’s essential to Establish economical logic from the beginning.
Commence by creating clean, uncomplicated code. Keep away from repeating logic and remove anything unwanted. Don’t select the most complex Answer if a straightforward one particular operates. Keep the features brief, concentrated, and simple to check. Use profiling equipment to locate bottlenecks—sites the place your code normally takes as well extensive to run or takes advantage of excessive memory.
Subsequent, check out your database queries. These generally slow points down over the code alone. Ensure that Every question only asks for the data you really have to have. Stay away from Find *, which fetches almost everything, and instead pick out particular fields. Use indexes to hurry up lookups. And avoid undertaking too many joins, In particular across huge tables.
For those who discover precisely the same data getting asked for many times, use caching. Shop the final results quickly employing applications like Redis or Memcached so that you don’t really need to repeat highly-priced functions.
Also, batch your database operations once you can. In place of updating a row one after the other, update them in teams. This cuts down on overhead and tends to make your app far more efficient.
Remember to examination with massive datasets. Code and queries that do the job fine with 100 records may well crash whenever they have to manage one million.
Briefly, scalable applications are speedy applications. Keep your code tight, your queries lean, and use caching when necessary. These methods support your software keep clean and responsive, whilst the load boosts.
Leverage Load Balancing and Caching
As your application grows, it's to deal with a lot more end users plus more visitors. If every thing goes via 1 server, it'll rapidly become a bottleneck. That’s where load balancing and caching are available. Both of these instruments support maintain your app fast, steady, and scalable.
Load balancing spreads incoming visitors across various servers. In lieu of just one server executing every one of the perform, the load balancer routes customers to different servers based on availability. This suggests no solitary server gets overloaded. If a person server goes down, the load balancer can send visitors to the Other folks. Resources like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to setup.
Caching is about storing data briefly so it may be reused immediately. When people request a similar facts once more—like a product web site or possibly a profile—you don’t have to fetch it within the database every time. You may serve it with the cache.
There are two popular varieties of caching:
one. Server-aspect caching (like Redis or Memcached) suppliers knowledge in memory for quickly access.
two. Shopper-aspect caching (like browser caching or CDN caching) suppliers static information near the user.
Caching lessens database load, enhances velocity, and helps make your application much more successful.
Use caching for things that don’t improve usually. And normally make sure your cache is up-to-date when details does modify.
Briefly, load balancing and caching are easy but strong applications. With each other, they assist your application deal with far more buyers, keep speedy, and recover from troubles. If you propose to grow, you will need both equally.
Use Cloud and Container Tools
To construct scalable programs, you require applications that let your app expand quickly. That’s where by cloud platforms and containers come in. They provide you overall flexibility, cut down set up time, and make scaling A lot smoother.
Cloud platforms like Amazon Net Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you need them. You don’t need to acquire hardware or guess potential ability. When targeted traffic boosts, you are able to include a lot more assets with only a few clicks or instantly making use of automobile-scaling. When site visitors drops, it is possible to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You are able to concentrate on building your application in lieu of running infrastructure.
Containers are A further critical Resource. A container deals your app and everything it really should operate—code, libraries, settings—into one device. This causes it to be straightforward to move your application among environments, from your notebook to your cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
When your application makes use of multiple containers, applications like Kubernetes allow you to control them. Kubernetes handles deployment, scaling, and Restoration. If one particular component of your application crashes, it restarts it immediately.
Containers also enable it to be very easy to separate portions of your app into products and services. You could update or scale areas independently, that is perfect for overall performance and trustworthiness.
In brief, applying cloud and container equipment means it is possible to scale fast, deploy quickly, and recover speedily when problems come about. If you would like your application to grow without having restrictions, start out utilizing these instruments early. They save time, lessen hazard, and enable you to keep centered on developing, not repairing.
Observe Every thing
When you don’t monitor your application, you gained’t know when points go wrong. Monitoring will help the thing is how your application is executing, place challenges early, and make much better choices as your application grows. It’s a critical part of developing scalable programs.
Start out by monitoring basic metrics like CPU usage, memory, disk Area, and response time. These let you know how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic can help you gather and visualize this info.
Don’t just keep an eye on your servers—watch your app too. Keep an eye on how long it will take for consumers to load webpages, how often mistakes take place, and in which they take place. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Create alerts for crucial difficulties. As an example, Should your response time goes above a Restrict or simply a company goes down, you need to get notified immediately. This helps you take care of challenges rapid, typically ahead of consumers even discover.
Checking is likewise check here valuable if you make adjustments. In the event you deploy a completely new element and see a spike in mistakes or slowdowns, you can roll it again just before it leads to serious problems.
As your app grows, visitors and information maximize. Devoid of monitoring, you’ll pass up signs of trouble until eventually it’s also late. But with the right instruments in place, you continue to be in control.
To put it briefly, monitoring allows you maintain your application reputable and scalable. It’s not just about recognizing failures—it’s about understanding your technique and making sure it really works well, even stressed.
Final Feelings
Scalability isn’t only for major organizations. Even compact apps will need a strong foundation. By building very carefully, optimizing sensibly, and using the appropriate tools, it is possible to Establish apps that increase smoothly without having breaking stressed. Start tiny, Imagine large, and Create good.